What is an algorithm?
A closer look at an important concept in modern technology
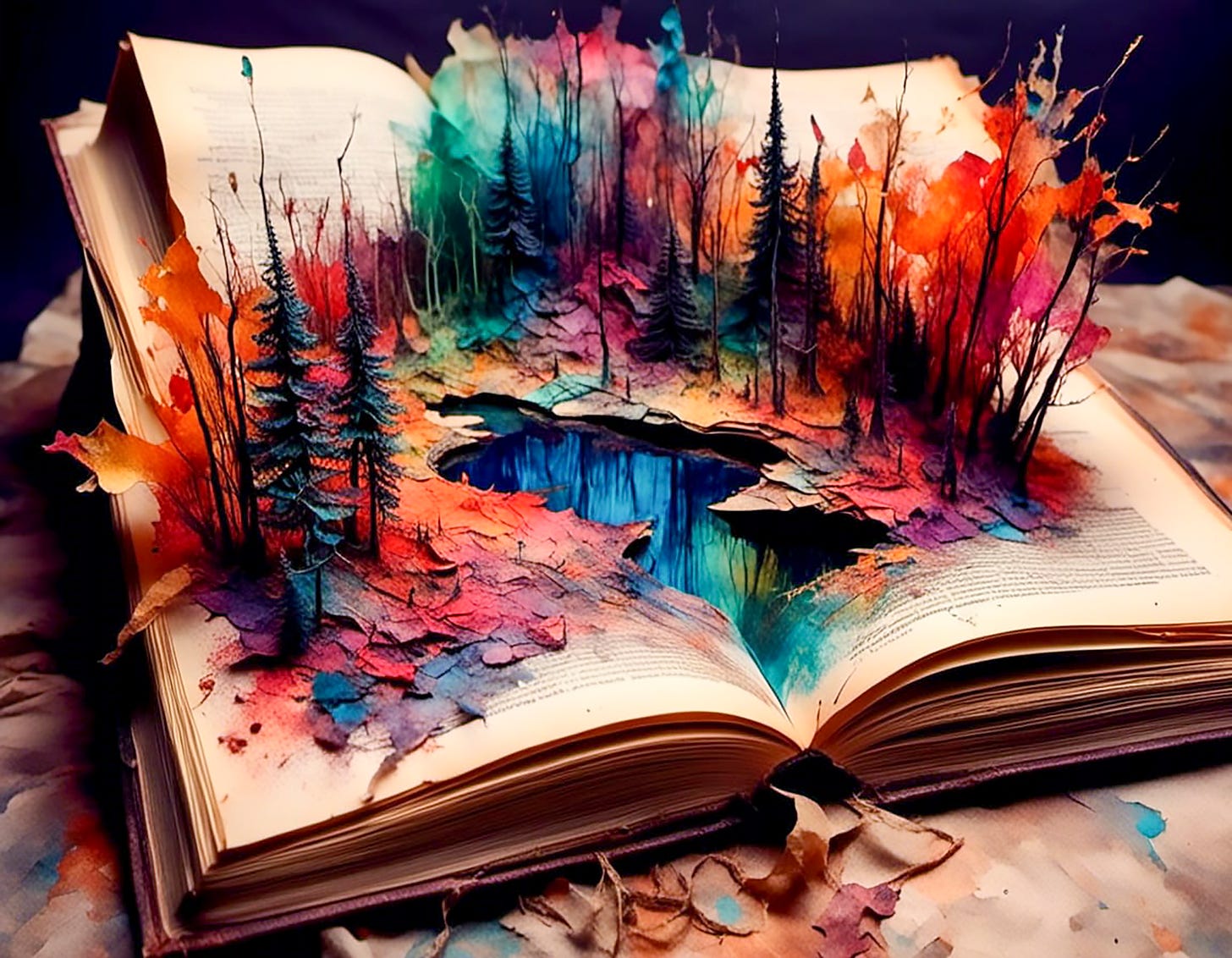
TL;DR
This newsletter provides a simple explanation of algorithms. It looks at what they are from both a technical and sociological point-of-view.
Here are the key takeaways:
On its face, an algorithm is an instruction about how to process given inputs that is interpreted and executed by a computer to produce an output.
Traditional algori…
Keep reading with a 7-day free trial
Subscribe to The Cyber Solicitor to keep reading this post and get 7 days of free access to the full post archives.